Screen-shot of output
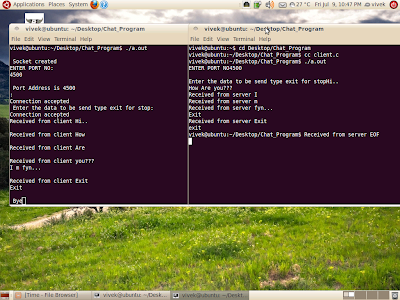
Program
server.c
#include "stdio.h"
#include "sys/types.h"
#include "netinet/in.h"
#include "string.h"
#include "sys/socket.h"
#include "stdlib.h"
#include "unistd.h"
main()
{
int sd,i,len,bi,nsd,port;
char content[30];
struct sockaddr_in ser,cli;
if((sd=socket(AF_INET,SOCK_STREAM,IPPROTO_TCP))==-1)
{
printf("\n Socket problem");
return 0;
}
printf("\n Socket created\n");
bzero((char*)&cli,sizeof(ser));
printf("ENTER PORT NO:\n");
scanf("%d",&port);
printf("\n Port Address is %d\n:",port);
ser.sin_family=AF_INET;
ser.sin_port=htons(port);
ser.sin_addr.s_addr=htonl(INADDR_ANY);
bi=bind(sd,(struct sockaddr *)&ser,sizeof(ser));
if(bi==-1)
{
printf("\n bind error, port busy, plz change port in client and server");
return 0;
}
i=sizeof(cli);
listen(sd,5);
nsd = accept(sd,((struct sockaddr *)&cli),&i);
if(nsd==-1)
{
printf("\ncheck the description parameter\n");
return 0;
}
printf("\nConnection accepted");
if(fork())
{
printf("\n Enter the data to be send type exit for stop:\n");
scanf("%s",content);
while(strcmp(content,"exit")!=0)
{
send(nsd,content,30,0);
scanf("%s",content);
}
send(nsd,"exit",5,0);
}
else
i = recv(nsd,content,30,0);
while(strcmp(content,"exit")!=0)
{
printf("\nReceived from client %s\n",content);
i=recv(nsd,content,30,0);
}
while(strcmp(content,"exit")!=0)
{
printf("\n Received from client %s\n",content);
i=recv(nsd,content,30,0);
}
printf("\n Bye");
send(nsd,"EOF",4,0);
close(sd);
close(nsd);
return 0;
}
client.c
#include "stdio.h"
#include "sys/types.h"
#include "netinet/in.h"
#include "string.h"
#include "sys/socket.h"
#include "stdlib.h"
#include "unistd.h"
int main()
{
int sd,con,port,i;
char content[30];
struct sockaddr_in cli;
if((sd=socket(AF_INET,SOCK_STREAM,IPPROTO_TCP))==-1)
{
printf("\n Socket problem");
return 0;
}
bzero((char*)&cli,sizeof(cli));
cli.sin_family = AF_INET;
printf("ENTER PORT NO");
scanf("%d",&port);
cli.sin_port=htons(port);
cli.sin_addr.s_addr=htonl(INADDR_ANY);
con=connect(sd,(struct sockaddr*)&cli,sizeof(cli));
if(con==-1)
{
printf("\n connection error");
return 0;
}
if(fork())
{
printf("\nEnter the data to be send type exit for stop");
scanf("%s",content);
while(strcmp(content,"exit")!=0)
{
send(sd,content,30,0);
scanf("%s",content);
}
send(sd,"exit",5,0);
}
else
{
i=recv(sd,content,30,0);
while(strcmp(content,"exit")!=0)
{
printf("Received from server %s\n",content);
i=recv(sd,content,30,0);
}
send(sd,"exit",5,0);
}
close(sd);
return 0;
}
Download the Source Code
server.c
client.c
6 comments:
nice....
thank u.....
You are Welcome...
we cse students feel ur blog to be very useful..especially the lab programs... we also want some more networks lab programs and java programs ..because we find the programs given in class is very difficult and we don feel like studying it....if u could upload the other programs also it would be usefull for us... can u do it for us??
@Angelina Thank u for ur comments...I am the one who maintains this blog, I am struck with fever right now and that s y some programs are yet to be uploaded...I will upload the programs that I have as soon as possible...Everything will be uploaded soon from next week....
hi,can u send ns2 programs
We generally use OPNET IT guru academic edition for Network Simulation...The website itself contains the manual for performing simulations in our syllabus...
Anyway I will post the links soon...
Then, If u r using Network Simulator 2 in Linux platform, then I am afraid i can't help u now because I haven't laid my hands on it...
Post a Comment