Program Status: Tested, Difficulty level: Easy
Program written by Vivek Venkatesh G
Screen-shot of output:
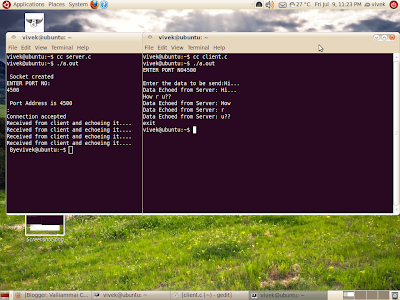
Program
server.c
#include "stdio.h"
#include "sys/types.h"
#include "netinet/in.h"
#include "string.h"
#include "sys/socket.h"
#include "stdlib.h"
#include "unistd.h"
main()
{
int sd,i,len,bi,nsd,port;
char content[30],buff[30],last_received[30];
struct sockaddr_in ser,cli;
if((sd=socket(AF_INET,SOCK_STREAM,IPPROTO_TCP))==-1)
{
printf("\n Socket problem");
return 0;
}
printf("\n Socket created\n");
bzero((char*)&cli,sizeof(ser));
printf("ENTER PORT NO:\n");
scanf("%d",&port);
printf("\n Port Address is %d\n:",port);
ser.sin_family=AF_INET;
ser.sin_port=htons(port);
ser.sin_addr.s_addr=htonl(INADDR_ANY);
bi=bind(sd,(struct sockaddr *)&ser,sizeof(ser));
if(bi==-1)
{
printf("\n bind error, port busy, plz change port in client and server");
return 0;
}
i=sizeof(cli);
listen(sd,5);
nsd = accept(sd,((struct sockaddr *)&cli),&i);
if(nsd==-1)
{
printf("\ncheck the description parameter\n");
return 0;
}
printf("\nConnection accepted");
i = recv(nsd,content,30,0);
send(nsd,content,30,0);
while(strcmp(content,"exit")!=0)
{
printf("\nReceived from client and echoeing it....");
i=recv(nsd,content,30,0);
send(nsd,content,30,0);
}
printf("\n Bye");
send(nsd,"EOF",4,0);
close(sd);
close(nsd);
return 0;
}
client.c
#include "stdio.h"
#include "sys/types.h"
#include "netinet/in.h"
#include "string.h"
#include "sys/socket.h"
#include "stdlib.h"
#include "unistd.h"
int main()
{
int sd,con,port,i;
char content[30];
struct sockaddr_in cli;
if((sd=socket(AF_INET,SOCK_STREAM,IPPROTO_TCP))==-1)
{
printf("\n Socket problem");
return 0;
}
bzero((char*)&cli,sizeof(cli));
cli.sin_family = AF_INET;
printf("ENTER PORT NO");
scanf("%d",&port);
cli.sin_port=htons(port);
cli.sin_addr.s_addr=htonl(INADDR_ANY);
con=connect(sd,(struct sockaddr*)&cli,sizeof(cli));
if(con==-1)
{
printf("\n connection error");
return 0;
}
if(fork())
{
printf("\nEnter the data to be send:");
scanf("%s",content);
while(strcmp(content,"exit")!=0)
{
send(sd,content,30,0);
scanf("%s",content);
}
send(sd,"exit",5,0);
}
else
{
i=recv(sd,content,30,0);
while(strcmp(content,"exit")!=0)
{
printf("Data Echoed from Server: %s\n",content);
i=recv(sd,content,30,0);
}
send(sd,"exit",5,0);
}
close(sd);
return 0;
}
Download Source Code
eserver.c
eclient.c
No comments:
Post a Comment